실행 결과 미리 보기
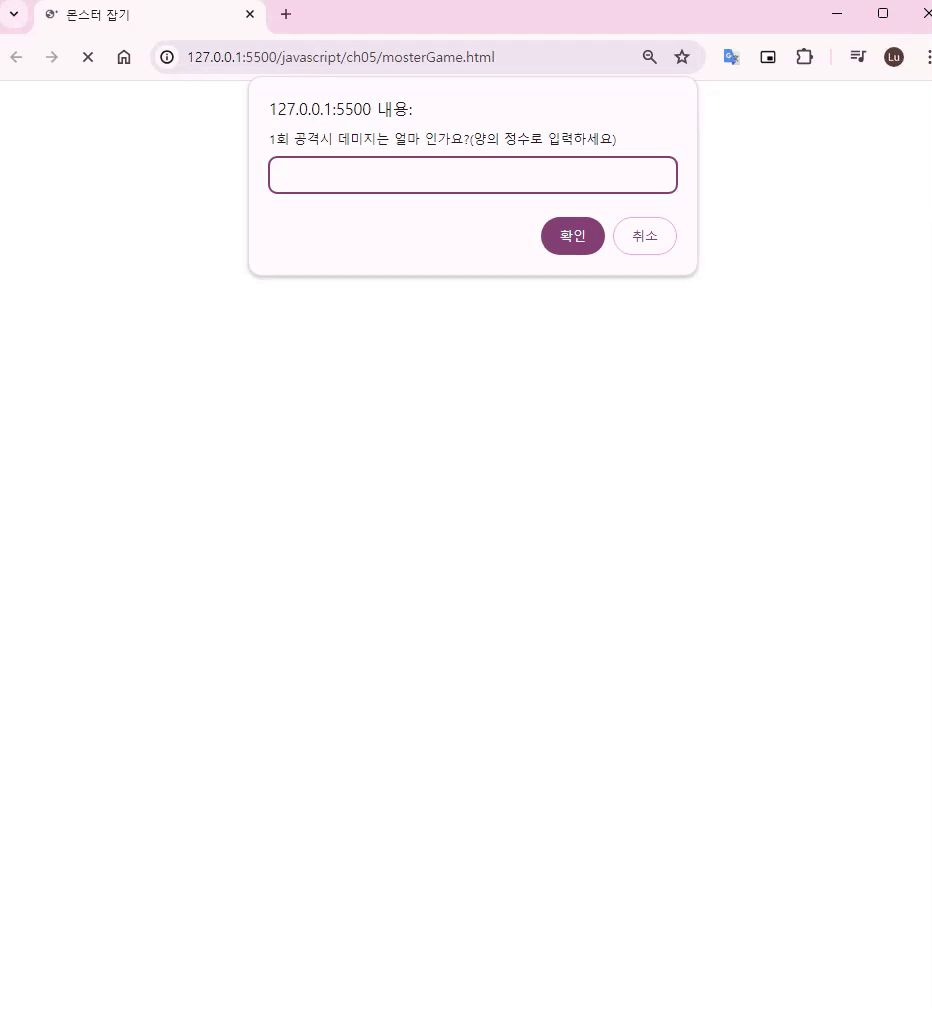
Today's
목표
1. DOM 메서드를 사용하여 새로운 노드를 생성하고 부모 노드에 포함하기
2. 사용자에게 데미지값을 prompt로 입력받아 과정/결과 출력
3. 데미지를 양의 정수로 입력 안 할 시, 게임 강제종료
추가 목표
1. Player 와 Monster 정보는 객체로 넣기
2. Player 와 Monster의 공격에 명중률을 넣어 공격이 실패하는 조건문 넣기
코드리뷰
01. Main
<div id="container">
<h1>몬스터잡기게임⚔</h1>
<div class="info">
<span id="player-info"></span>
<span id="monster-info"></span>
</div>
<div class="game-log"></div>
</div>
02. Player,Monster 객체 정보
//플레이어 정보
const player={
hp: 100,
attackDamage: Number(prompt('1회 공격시 데미지는 얼마 인가요?(양의 정수로 입력하세요)')), //사용자 데미지
aim: Math.floor(Math.random()*(100-70)+70), //사용자 명중률
}
//몬스터 정보
const monster={
hp: 100,
attackDamage: Math.floor(Math.random()*(20-10)+10), //몬스터 공격 데미지
aim: Math.floor(Math.random()*(60-50)+50), //몬스터 명중률
}
//플레이어와 몬스터 정보 출력하기
const info = document.querySelector('.info');
info.querySelector('#player-info').innerHTML =
`[Player]
HP: ${player.hp}
AttackDamage: ${player.attackDamage}
Aim: ${player.aim}
`;
document.querySelector('#monster-info').innerHTML =
`[Monster]
HP: ${monster.hp}
AttackDamage: ${monster.attackDamage}
Aim: ${monster.aim}
`;
03. 게임 시작
//게임 시작 문구
const container = document.querySelector('#container');
const start = document.createElement('h2');
start.textContent = "몬스터 잡기 게임을 시작합니다!";
container.insertBefore(start,info); //특정 위치에 노드삽입
04. 플레이어 턴
.player-log{
height: 50px;
margin-bottom: 5px;
padding: 0 20px 0 20px;
border-radius: 10px;
background-color: rgb(105, 140, 255);
line-height: 50px;
text-align: right;
position: relative;
left: 460px;
}
- Player와 Monster 의 Status 말풍선을 구분하고
Player는 오른쪽, Monster는 왼쪽으로 정렬해서 보이기
if(Math.random() * 100 < player.aim){ //100중에 aim이 크다면 적중
monster.hp -= player.attackDamage;
if(monster.hp < 0){
monster.hp = 0;
}
const playerLog01 = document.createElement('p');
const playerLog02 = document.createElement('p');
playerLog01.className = 'player-log';
playerLog02.className = 'player-log';
playerLog01.textContent = `플레이어의 ${attackCnt}번째 공격이 성공했습니다.`;
playerLog02.textContent = `몬스터의 남은 HP는 ${monster.hp}입니다.`
gameLog.appendChild(playerLog01);
gameLog.appendChild(playerLog02);
//몬스터 HP 0일때 다음 몬스터턴으로 안넘어가게 나가기
if(monster.hp === 0){
break;
}
}
else{
const playerLog03 = document.createElement('p');
playerLog03.className = 'player-log';
playerLog03.textContent = `플레이어의 ${attackCnt}번째 공격이 빗나갔습니다`;
gameLog.appendChild(playerLog03);
}
05. 게임 종료
let endText = player.hp > 0 ? '플레이어의 승리입니다!':'플레이어가 사망했습니다!'
const end = document.createElement('h2');
end.textContent = endText;
container.appendChild(end); //맨마지막에 노드삽입
- 삼항 연산자를 사용해서 player의 HP에 따라 나오는 문구 입력하기
else{
alert('공격 데미지를 양의 정수로 입력하지 않았습니다. 게임을 종료합니다.');
history.go(0); //0이면 새로고침/ -1을 넣으면 이전페이지로
}
- hisstory.go(1)로 alert이 종료되고 바로 새로고침될 수 있게 함
= hisstory객체는 캐쉬가 가능한 경우 입력된 양식이 삭제되지 않고 유지되기 때문에 form 태그 등에 자주 사용됨
history.back()
현재 페이지의 오직 한단계 이전페이지로 이동합니다.
histroy. back()은 histroy.go(-1)과 동일하게 한페이지 전으로 가지만 histroy.go(-1)이 보편적으로 사용됩니다
histroy.go()
이전 또는 이후 페이지의 이동이 가능합니다.
전달할 인자에 숫자를 넣어 원하는 만큼 뒤로 이동할 수 있게 됩니다
history.forward()
이 방법을 사용할 경우 이후 페이지인 다음 페이지로 이동합니다. history.forward() 와 history.go(1)과 동일합니다
만약 다음으로 이동할 페이지가 없는 경우 동작하지 않습니다
마무리
보완해야 할 점
1. p태그 말고 li태그로 player와 monster의 log 출력하기
2. 채팅방 말풍선 효과처럼 한 번에 출력이 아닌 순차적으로 Log가 뜰 수 있게
추후 공부 할 내용
1. DOM element를 삽입하는 방법 개념 정리하기
append() / prepend() / insertBefore() / insertAdjacentElement()
2. className과 classList 개념 정리하기
3. hisstory 객체 개념 정리하기
'DEVELOPMENT > JavaScript' 카테고리의 다른 글
[JS] 실습예제 (3) | 2024.04.26 |
---|---|
[JS] 데이터 타입 (0) | 2024.04.24 |
[JS] 변수 (0) | 2024.04.24 |
[JS] 크리스마스 카운트다운 (2) | 2024.04.24 |
[JS] 간단 회원가입 Form 만들기 (3) | 2024.04.24 |